How To Beep In Python - 5 Simple Ways
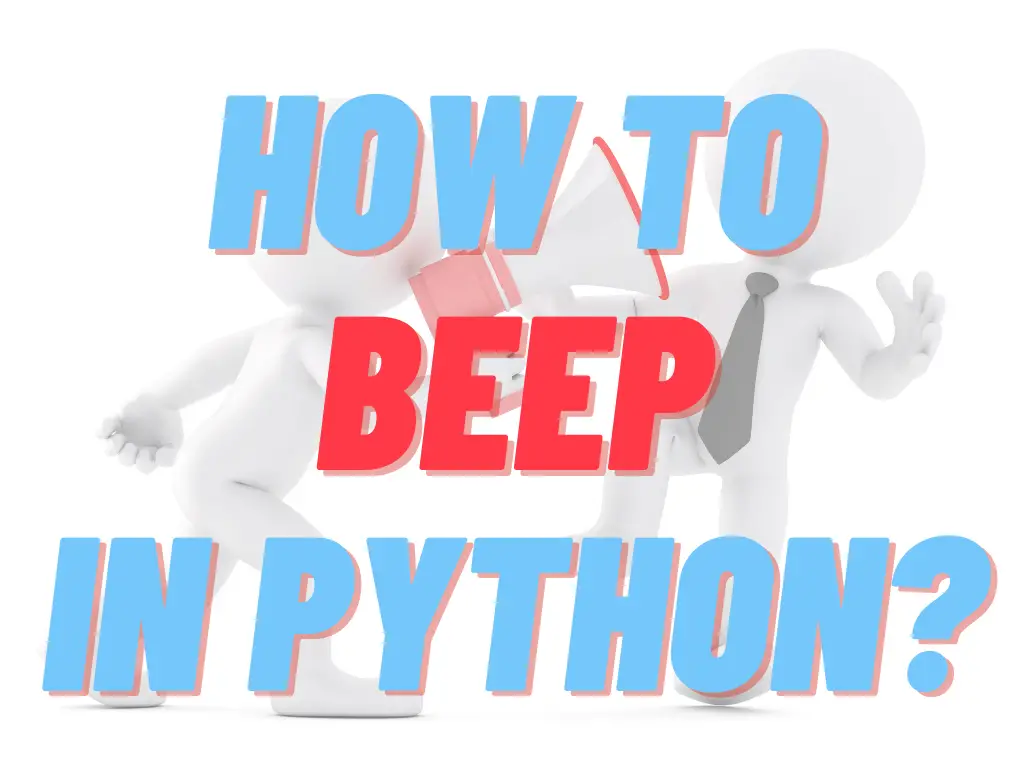
In this tutorial I will show you 5 simple ways to generate a beeping sound in Python.
To generate a beeping sound in Python you have the following options:
- Use the bell character on the terminal
- Use AppKit to play MacOS system sounds
- Use winsound to play Windows system sounds
- Use pygame to play custom sound files
- Use simpleaudio to play custom sound files
- Use the beepy package
To put it in a more a pythonic way: how to make your machine go PING! (HINT: check the end of the article if you don’t get the reference.)
1. Using The Bell On The Terminal
There is a so-called bell character that you can use to issue a warning on a terminal. It is a nonprintable control code character, with the ASCII character code of 0x07 (BEL). You can trigger it by simply sending it to the terminal (note the backslash):
print('\a')
This is probably the most simple way of sounding a beep, though it is not 100% guaranteed to work on all systems. Most UNIX-like operating systems like macOS and Linux will recognize it, but depending on the current settings the bell might be muted or be represented as a flash on the screen (visual bell).
2. Using AppKit.NSBeep On MacOS
If you’re on a Mac, you can tap into the Objective-C libraries to generate a sound.
First you’ll need to install the PyObjC library:
pip install -U PyObjC
Then you can simply use the AppKit
interface the ring the default system sound, like so:
import AppKit
AppKit.NSBeep()
3. Using winsound On Windows
On Windows operating systems you can use the winsound
library.
winsound
needs no installation it is a builtin module on windows, so you should be able to access it by default
windound
a have a handy Beep
API, you can even choose the duration and the frequency of the beep. This is how you generate a 440Hz sound that lasts 500 milliseconds:
import winsound
winsound.Beep(440, 500)
You can also play different windows system sound effects using the PlaySound
method:
import winsound
winsound.PlaySound("SystemExclamation", winsound.SND_ALIAS)
The same API can be used to play custom sound files using the SND_FILENAME
flag instead of SND_ALIAS
:
import winsound
winsound.PlaySound("beep.wav", winsound.SND_FILENAME)
4. Playing Sound Files With pygame
Pygame is a modular Python library for developing video games. It provides a portable, cross-platform solution for a lot of video game and media related tasks, one of which is playing sound files.
To take advantage of this feature, first you’ll need to install pygame:
pip install pygame
Then you can simply use the mixer
the play an arbitrary sound file:
from pygame import mixer
mixer.init()
sound=mixer.Sound("bell.wav")
sound.play()
Just like with the previous solution, you’ll need to provide you own sound file for this to work. This API supports OGG and WAV files.
5. Playing Sound Files With Simpleaudio
Simpleaudio is a cross-platform audio library for Python, you can use it to play audio files on Windows, OSX and Linux as well.
To install the simpleaudio package simply run:
pip install simpleaudio
Then use it to play the desired sound file:
import simpleaudio
wave_obj = simpleaudio.WaveObject.from_wave_file("bell.wav")
play_obj = wave_obj.play()
play_obj.wait_done()
6. Use Package Made For Cross-Platform Beeping - Beepy
If you want a ready-made solution you can check out the beepy
package. Basically it’s a thin wrapper around simpleaudio, that comes bundled together with a few audio files.
As always, you can install it with pip:
pip install beepy
And then playing a beep sound is as simple as:
import beepy
beep(sound="ping")
Summary
As you can see there are several different ways to go about beeping in Python, but which one is the best?
If you just want a quick and dirty solution I’d recommend trying to sound the terminal bell. If you want something more fancy or robust I’d go with winsound on windows or AppKit on a Mac. If you need a cross-platform solution your best bet will be using simpleaudio or pygame, to get a custom sound file played.
Congratulations, now you’ll be able to turn your computer into “The Machine That Goes PING”.