Working with Python Lists
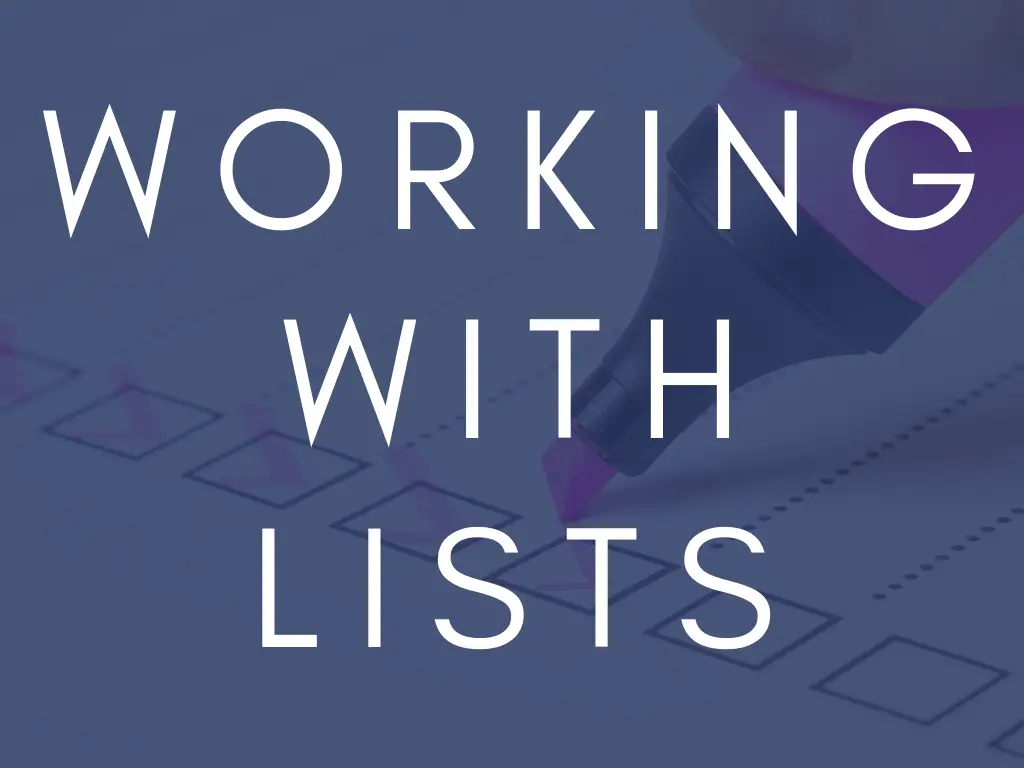
Lists are one of the builtin data types of the Python programming language, they can be used to store a collection of values. In this tutorial I will share a few common patterns - recipes if you like - to help you better understand and work more effectively with lists.
Reversing a list
There are quite a few different ways to reverse a Python list, each has it’s advantages and drawbacks:
Using the reversed builtin
The reversed
builtin takes a list
as it’s argument and returns a new list
, containing the same elements, but in increasing order. The original list will be left intact, reverse
does not change it.
Example usage:
l=[1,2,3,4,5]
print(list(reversed(l))
output:
[5,4,3,2,1]
Why is it necessary to convert the return value to a list on the second line? Because actually, the reversed
function does not returns a list
, but an iterator. Iterators work very similarly to lists, but they have a feature called lazy evaluation, meaning that the elements are computed on-the-fly, so you can loop over them just like you would with a list, but you cannot access an element randomly (unlike lists, iterators are not indexable).
So this:
l=[1,2,3,4,5]
print(list(reversed(l))
would just print something like:
<listreverseiterator object at 0x7f89b3467d90>
However, this:
l=[1,2,3,4,5]
for i in reversed(l):
print(i)
would work as expected:
5
4
3
2
1
Reversing a list with the slicing operator
The slicing operator (square brackets) helps you to select certain element from a list. It takes three parameters:
- start of the slice
- end of the slice
- step
The parameters are separated by colons (:
).
For example, to get every second element from a list, starting from the third and up until the sixth, you can write:
l=[1,2,3,4,5,6,7,8,9,10]
print(l[3:6:2])
This will print:
[4,6]
Now, how can we use it to reverse our list? Negative values are allowed in place of the 3rd parameter, and they will mean that we’re stepping backwards on the slice. So the trick is to use -1
as the step.
The first and second parameters can be omitted, and in this case they will default to the start and the end of the whole list.
So to get the entire list, but reversed, we can use the slice operator like this:
l=[1, 2, 3]
print(l[::-1])
The result should be:
l=[3, 2, 1]
Reversing a list in-place
If you don’t mind modifying the original list, you can stick with the list’s reverse
method. This method returns None
, but rearranges the original lists (so the elements will be in increasing order).
l=[1,2,3,4,5]
l.reverse()
print(l)
Sorting lists
Python provides two basic ways to sort a list: the sorted
builtin and the list
object’s sort
method.
Creating a new - sorted - list
The sorted
builtin takes an iterable and returns a new, sorted one, without modifying the original instance.
Example:
l = 3, 1, 7, -2
print(list(sorted(l)))
output:
[-1, 1, 3, 7]
Note: The conversion to list is needed in this case, because sorted returns an iterator, not a list. Print would not print the values of the iterator, so we need a list here, but if you just need to loop over the result, the list
conversion can be omitted.
Sorting lists in place
If you do need the original list, it is recommended to just modify it by sorting it in-place, using the lists sort
method. sort
returns None
, but changes the original list by rearranging the elements.
Example:
l = 3, 1, 7, -2
l.sort()
print(l)
output:
[-1, 1, 3, 7]
Sorting a tuple
The sorted
method can be used on tuples as well:
t = 3, 1, 7, -2
print(sorted(t))
output:
[-1, 1, 3, 7]
Note, that the sorted method always returns a list. So if you want a sorted tuple, you’ll have to convert it to the proper data type:
t = 3, 1, 7, -2
print(tuple(sorted(t)))
output:
(-1, 1, 3, 7)
Sorting a tuple without creating a new instance
Python tuples are immutable, meaning you cannot modify them, so it is not possible to sort them in-place. You must use sorted, as tuples do not have a sort
method like lists do.
This will result in an error:
l = 3, 1, 7, -2
l.sort()
Output:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'tuple' object has no attribute 'sort'