How To Import From Another Folder In Python?
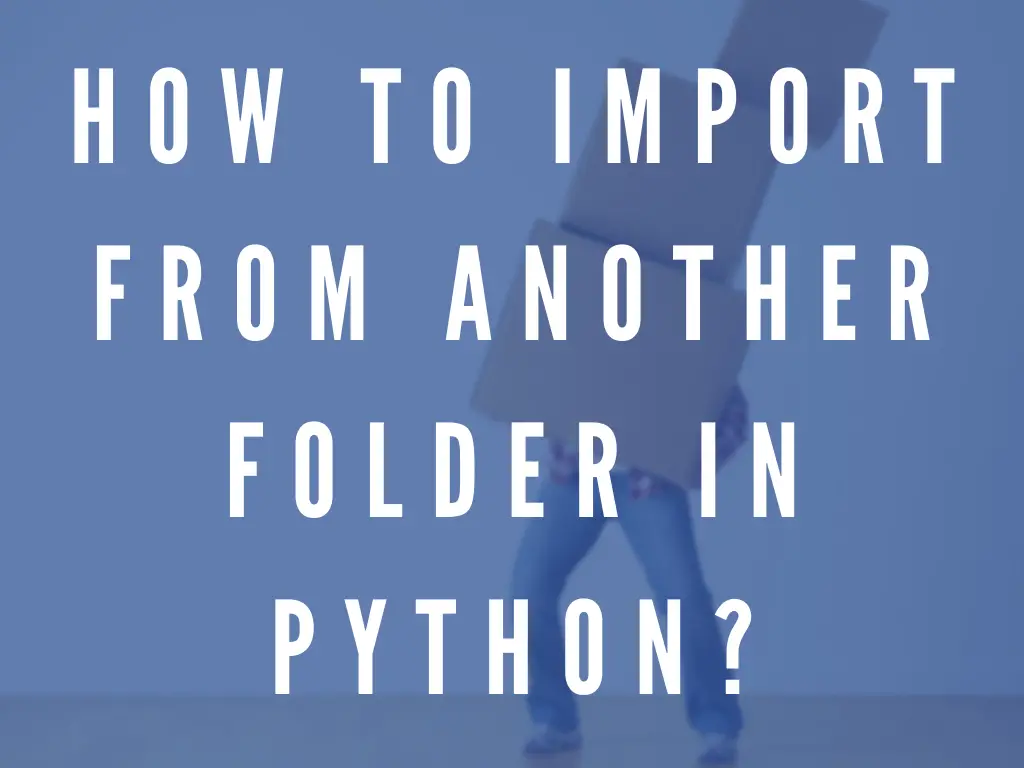
Python has a bit of a unique import system, if you’re trying to import files from a directory outside of your package, you might run into some trouble.
By default Python does not allow importing files from arbitrary directories, but there is a workaround: you can add the directory to your PYTHONPATH
env var or insert it into the sys.path
variable.
In this short tutorial, I’ll show you how to do this, explain why it’s not a good idea, and show some better ways to fix this problem.
1. Add The Folder To Your PYTHONPATH
Environment Variable
When you try to import
something in Python, the interpreter will first look for a builtin module.
If no builtin module is found, Python falls back to searching in the current working directory.
If the module is not found there, the Python engine will try all the directories listed in the sys.path
system variable. When starting the script, this variable is initialized from the PYTHONPATH
environment variable.
To add an extra import source folder, before running your script, run one of the following snippets (depending on your OS):
On Linux Or MacOS
export PYTHONPATH=/path/to/file/:$PYTHONPATH
Windows
set PYTHONPATH=C:\path\to\file\;%PYTHONPATH%
As you can see PYTHONPATH
contains a list of directories, separated by :
. We inserted /path/to/file/
(or \path\to\file
) at the beginning of the string, so this will be the first place where Python will look for files to import.
2. Add The Folder To sys.path
If you cannot or do not want to modify your env vars, there is an alternative: you can also directly modify the sys.path
variable at runtime.
You can insert your directory at the beginning of the list of the available import paths by adding these two lines of code to your Python script (before the import statement):
import sys
sys.path.insert(1, '/path/to/file/')
This will have exactly the same effect as modifying your PYTHONPATH
.
Troubleshooting: Make Sure You Have an __init__.py
File In The Containing Folder
If you still have issues importing the files you want, make sure that the containing directory has an __init__.py
file. This is used to mark the folder as a valid Python package. If your folder does not have one, you won’t be able to import files from there, as the Python interpreter only allows importing from packages, and not from standalone files.
Disclaimer: Probably There’s A Better Way To Solve Your Problem
You might notice, that Python does not make importing files from arbitrary folders easy, and there is a good reason for that. Tinkering with sys.path
or PYTHONPATH
is probably not a good idea. In some cases it can be used as a quick hack to test something, but definitely should not be used in production.
It makes your system quite brittle, and can also cause serious security issues. There are better ways to go about it:
1. Simply Include The File You Want To Import In Your Project.
Just copy/move the file into a subdirectory in your working folder. Maybe create a submodule for it.
2. Install It In A Proper Location
If it is part of a third party package, chances are you can just install it with pip
. Pip - the default Python package manager - will copy it to its proper place: some system-specific directory (like /usr/lib/python/
), which is already contained in your PYTHONPATH
, so the Python interpreter can automatically find it without the need to tweak your env vars.
3. Create Your Own Package
If it’s not 3rd-party code, but only loosely related to your current project, or it’s something like a library of classes/functions that you reuse in multiple projects, then the best way would probably be to bundle it up and create your own package. That way you can treat it exactly like a 3rd party pip
package.