What's The Difference Between __str__ And __repr__ In Python?
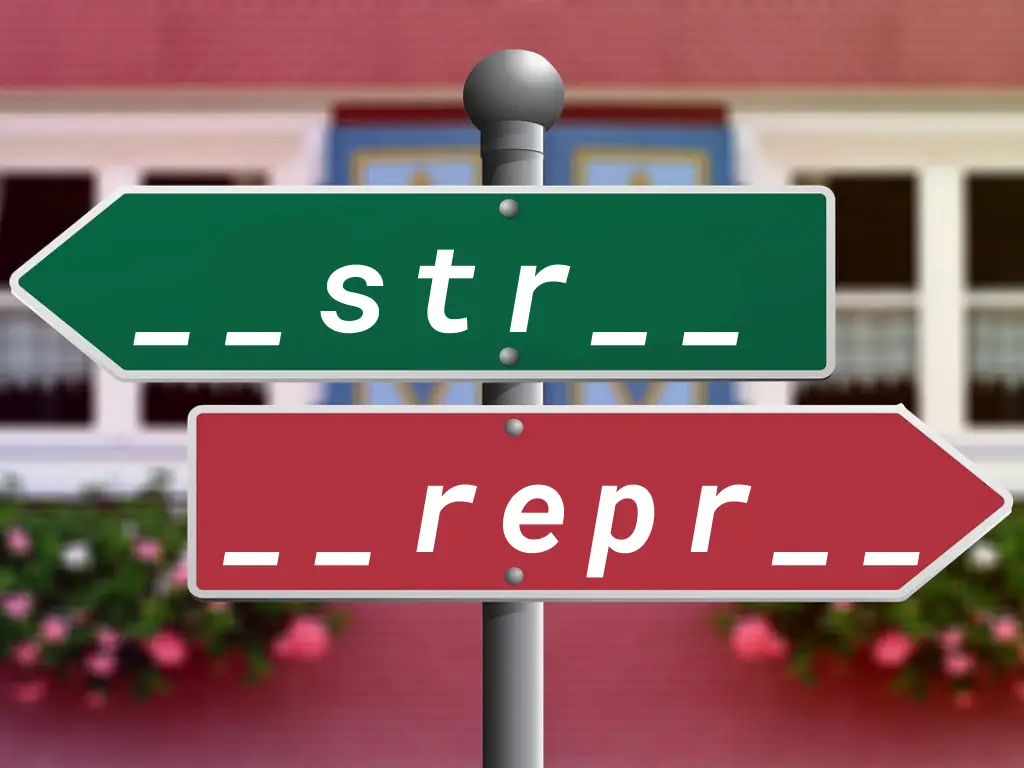
Python objects have two different string representations, namely __str__ and __repr__, in some cases these can return the same value, but there is an important difference between the two.
In Python __str__ is meant to return the object’s human-friendly representation, while __repr__ should return a valid python expression that can be used to recreate the object.
In the following sections, we’ll look at some examples, and I’ll show you how to implement these magic methods correctly for your own classes.
Magic Methods
Most of the “automagical” behavior - like comparision, conversion or object initialization - of Python classes are implemented as magic methods or the so-called dunder methods. The name comes from the double underscore naming conventions.
These methods are usually not called explicitly, but get triggered automatically in certain scenarios.
str() and repr() vs str() and repr()
A good example can be the __str__()
method, which gets called, whenever an object is passed to the str()
builtin, or it’s twin the repr()
builtin which calls the object’s __repr__()
dunder method.
Both return a kind of string representation of the given object, with a slight, but important difference between the two.
What’s The Goal of str()?
__str__()
is meant to return a human-readable, friendly, verbose representation of the object. Think of it as a string representation that’s suitable for the end-user of your Python program.
What Is repr() For?
In contrast __repr__()
should always return a unique representation of the object. If it is possible the return value should be a valid Python expression that can be used to recreate the original object.
In other words eval(repr(obj))
should equal obj
.
If this is not possible, then __repr__
should be a useful description of the object enclosed in triangular brackets - usually something like <ClassName instance_id>
Default Implementations
If a class does not implement a custom __str__
method, the default implementation will be used, which is just falling back to __repr__
on string conversion.
For this reason it is always a good idea to implement a working, meaningful __repr__
dunder method for your classes.
Containers And Recursive Data Structures
It might be surprising at first, so it’s good to note here that all containers’ and recursive data structures’ __str__
method uses the contained objects’ __repr__
method instead of its __str__
- for readability reasons.
Closing Thoughts
The key takeaway points from this short tutorial:
__str__
is for humans, it should be readable__repr__
should be a valid Python expression if possible, or a meaningful, unique representation of the object.- You should always implement a
__repr__
method for your classes - You can optionally implement a
__str__
method, if you think that a nice human-readable representation is necessary