Python Project Ideas
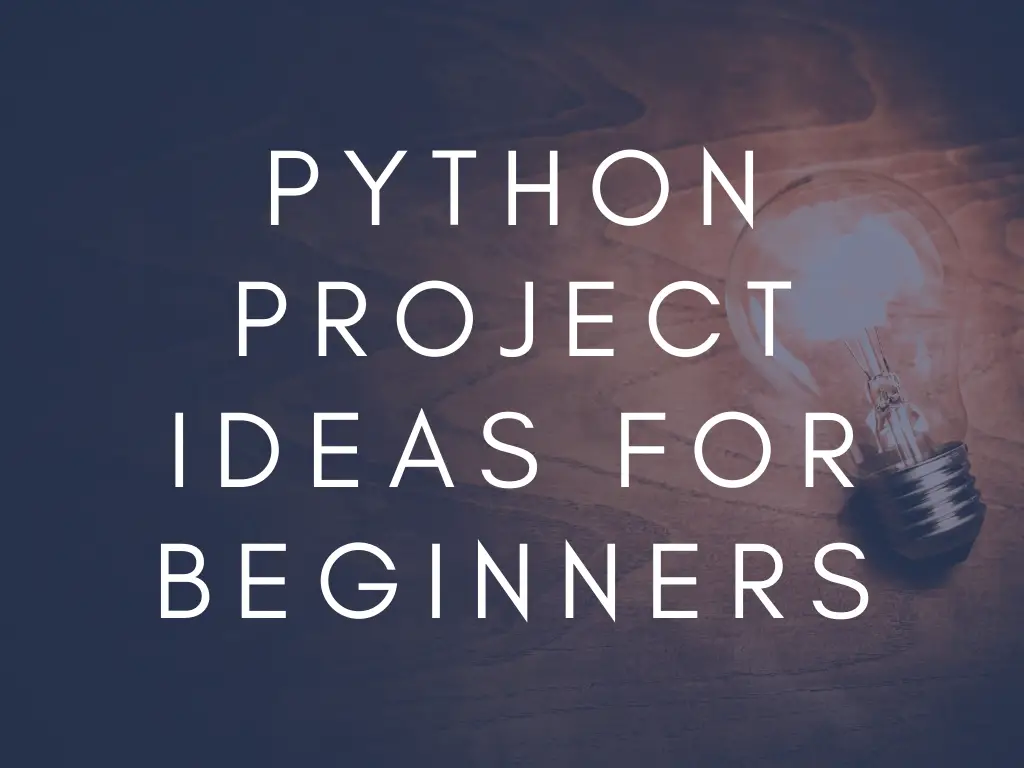
In this article I collected a few interesting project ideas for you to improve your Python coding skills. All ideas come with a follow-along video and copyable source-code.
Table of Contents
Beginner Project Ideas
Beginner Python Project 1: Body Mass Index Calculator
Idea
Build a script that asks the user’s body weight and height and prints his/her BMI value and classification. You can copy the BMI formula and the classification form Wikipedia.
Follow along video:
Source code:
def ask_user():
return (
float(input("Your height:")),
float(input("Your weight:"))
)
def get_bmi_index(height, weight):
return weight/height**2
def get_bmi_class(bmi):
classes = {
15: "Very severely underweight",
16: "Severely underweight",
18.5: "Underweight",
25: "Normal (healthy weight)",
30: "Overweight",
35: "Obese Class I (Moderately obese)",
40: "Obese Class II (Severely obese)",
float('inf'): "Obese Class III (Very severely obese)"
}
for limit, bmi_class in classes.items():
if bmi < limit:
return bmi_class
height, weight = ask_user()
bmi = get_bmi_index(height, weight)
bmi_class = get_bmi_class(bmi)
print('Your BMI is {}, you are classified as "{}"'.format(bmi, bmi_class))